Lists and Keys
In any application, at some point, you will end up having to deal with some kind of list. In javascript, a "List" is a collection of elements {}.
const myList = [
{
item: "banana"
},
{
item: "avocado"
},
{
item: "eggs"
}
];
When displaying lists on React/ReactNative, the elements of a list should have a unique key.
- The key MUST be unique between siblings;
This rule is very important because...
"Key is the only thing React uses to identify DOM Elements"
Mainly if your list will be filtered or sorted, using the wrong key may lead to errors.
Avoid using INDEX as key
It's not recommended to use the INDEX of the element as key, because it negatively impacts performance and the display data.
Let's take the index (starting on 1).
const myList = [
{
item: "banana"
},
{
item: "avocado"
},
{
item: "eggs"
}
];
...
myList.map(
(el, index) => {
return (
<View key={index}>
<Text>{el.item}</Text>
</View>
);
}
);
Consider removing the ID 2 (Acovado). In the new list, the element ID 2 will still exist, but now as "Eggs", because we are using the index of the list.
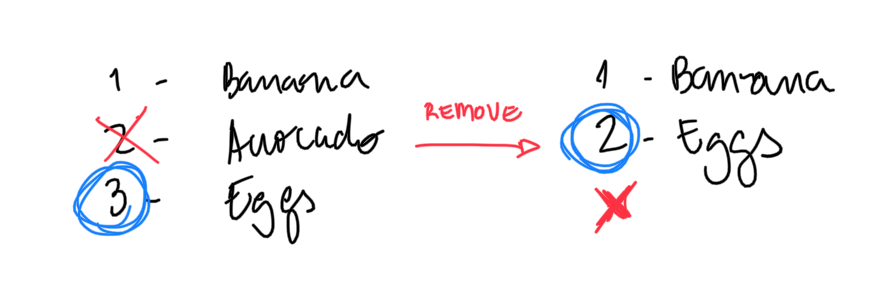
In this hypothetical example, let's say the ID will be a unique letter.
const myList = [
{
id: "B",
item: "banana"
},
{
id: "A",
item: "avocado"
},
{
id: "E",
item: "eggs"
}
];
...
myList.map(
(el, index) => {
return (
<View key={el.id}>
<Text>{el.item}</Text>
</View>
);
}
);
In this case, we will not face the problem of having the same ID after removing the "ID A"
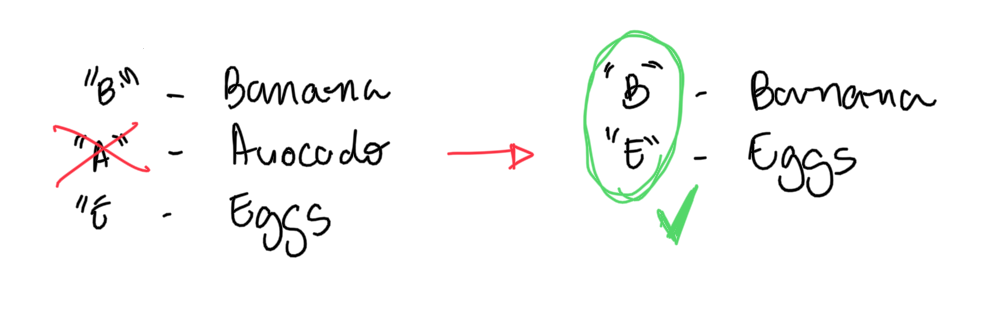
A real-world case
On my job, I was creating a Flalist for displaying a collection of NFTs. Because the backend was not ready and I was using the mocking data for development without ids, I decided to use a random number as a key for the list.
key={Math.random().toString()}
It was an OK implementation for dev, but the keys were dynamically generated in each render, and the user had a expand/collapse button. When the "See All" button was pressed, the random function generates new ids and the React was considering new items, causing some weird flicker effect.
Once the backend sent the correct data with UUID, which are unique and consistent between renders, the flicker problem went away.
Using the wrong key may break your app or display some wrong data. So choose your keys wisely ;-)